12 Java Programming Challenges for Beginners and Strategies to Overcome Them with Codeyoung
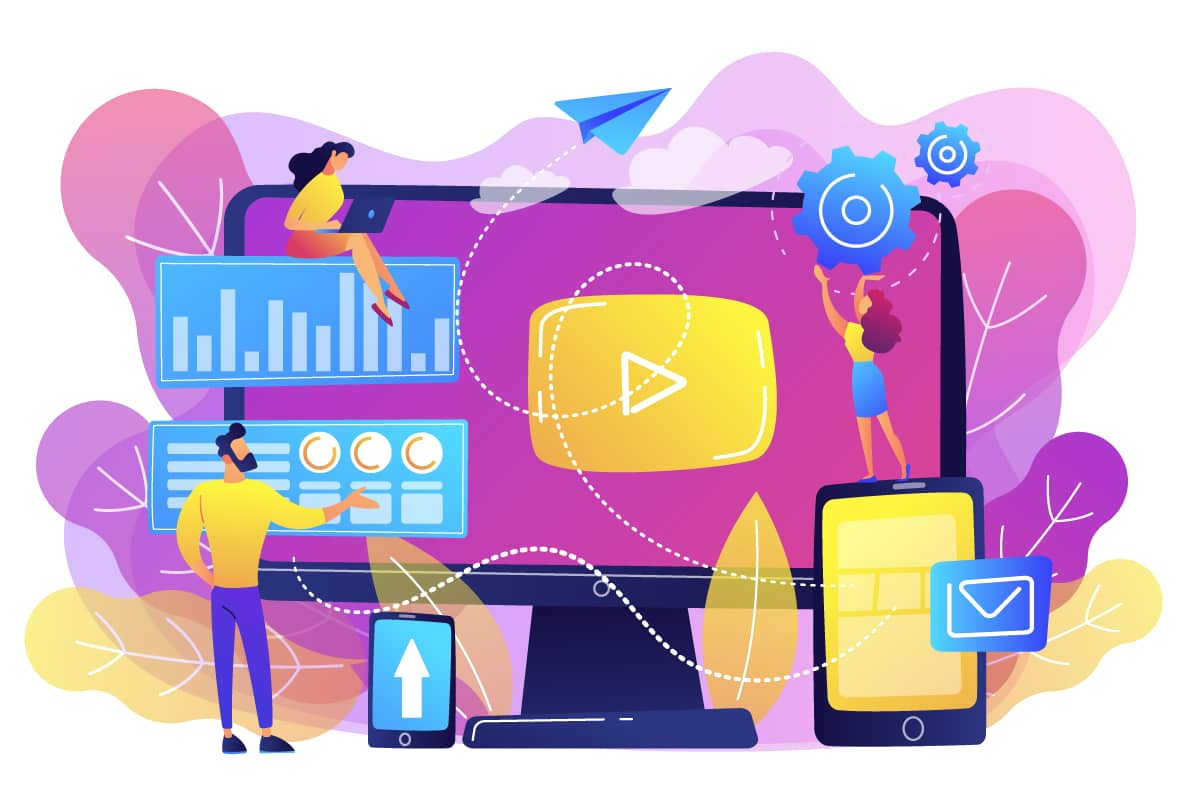
The world currently employs Java (object-oriented programming) as one of its most commonly utilized programming languages. Java remains essential for developers since the language brings versatility to developers along with platform independence and operational flexibility across numerous applications. Java mastery provides access to a vast array of professional prospects that enable you to build backend systems together with dynamic applications. The Java programming language includes unique challenges that present difficulties, especially to starting programmers.
Java presents numerous difficulties for beginner programmers because OOP specification and data structure solid understanding, together with complex syntax error management, are critical aspects of the language. The way to master an object-oriented programming language, i.e., Java, becomes exceedingly difficult because of these learning obstacles. Many learners seek structured support through java programming practice exercises or a java programming online course to ease this process. But fear not! The following blog presents 12 standard difficulties that novice Java programmers commonly encounter. The blog combines java coding problems and solution with classes, offering applicable remedy methods that students can implement to handle these issues successfully.
Throughout the blog, we will demonstrate that the right approach, combined with the necessary resources, enables you to face these obstacles with self-assurance while becoming proficient in Java development. Whether you're engaging in java coding challenges or working through basic Java programming codes, understanding the root problems is key. The following discussion examines the difficulties and solution methods that will support your learning progress.
Java Code Challenges
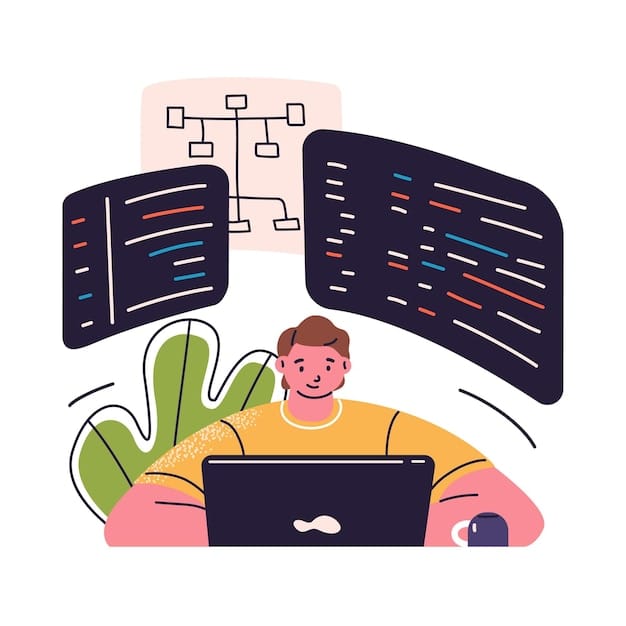
Practicing Java coding and java programming practice exercises on a regular basis, together with diverse programming tasks, leads to mastering the language effectively. Participating in practical Java coding challenges enables you to become a more competent problem solver while deepening your understanding of fundamental programming theory. A collection of 12 starting java coding projects exists, which teaches students fundamental programming principles that increase in complexity through essential syntax understanding up to algorithmic sophistication.
The coding challenges in Java you will encounter in this training have specifically been chosen to develop logical reasoning alongside algorithm development and efficient code practices. These exercises evaluate your Java code writing competencies while prompting you to develop sophisticated solutions. The designed challenges will lead students through essential Java programming skills, including loop and array understanding and object-oriented concepts for building confident Java code abilities. From basic Java programming codes to more advanced techniques, each challenge strengthens your grasp of structured problem-solving. The time has come to face these challenges that will help you develop your programming skills!
Word Reversal
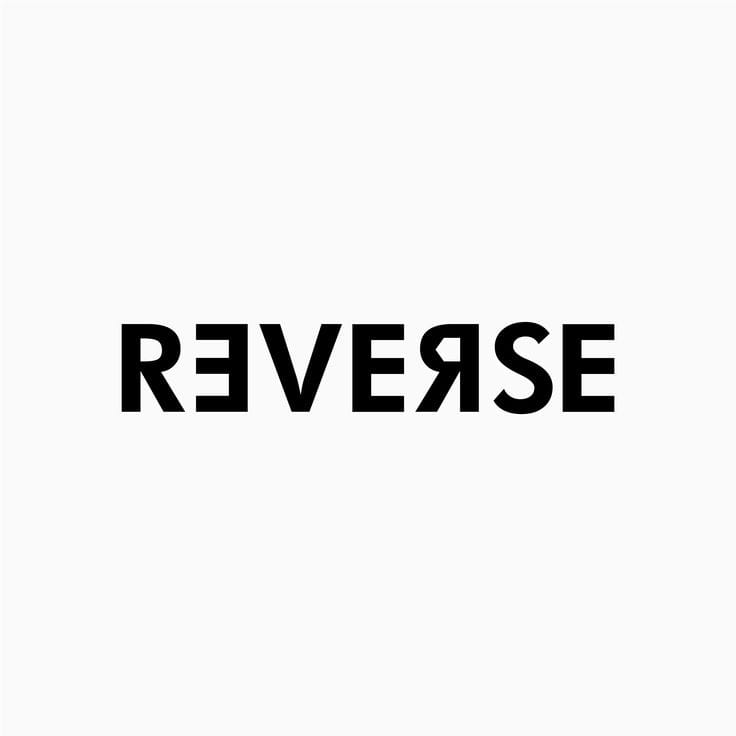
Challenge: Develop an application written in Java that transforms a specified word into its reversed character order. This task forms part of foundational java coding challenges that help sharpen beginner logic and syntax.
Concepts Covered:
String manipulation: This lesson teaches efficient methods to alter and modify strings in the Java programming language, which forms the basis of many Java programming challenges.
Loops and conditionals: According to the challenge, you need to use loops along with conditionals in order to process string data and handle various test scenarios together with boundary conditions. These elements are essential for tackling java coding problems and solution with classes in more advanced scenarios.
Strategy:
A Java string reversal requires the efficient approach of using the StringBuilder class. The reverse() method of this class helps perform operations on mutable strings while providing easy string modifications. The use of StringBuilder prevents the creation of several string objects that slow down performance, making it a preferred approach even in basic Java programming codes.
Through this exercise, you can experience input/output operations, which enable you to become comfortable both reading console input numbers and printing Java outputs. A basic structure for this task would be:
The program requests user input of a word.
You should transform the string data into a StringBuilder object.
Apply the reverse() method to the string for reversion.
Print the reversed string.
Using this approach, you can develop an efficient solution that is also simple to execute and improve your skills in string manipulation techniques as well as coding logic.
Find the Word
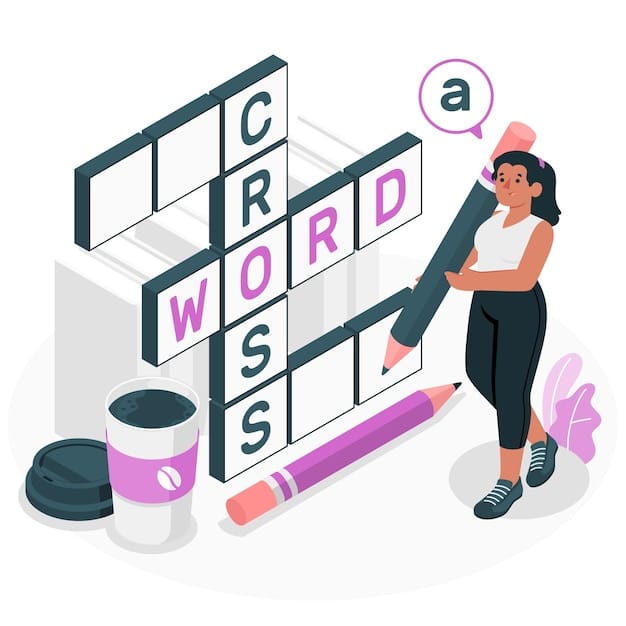
Challenge: Develop an application that detects the position of target words found in textual sentences. This task forms part of beginner-friendly java coding challenges that help refine string search and manipulation skills.
Concepts Covered:
String search methods: You will learn about Java’s standard search methods for strings by exploring the indexOf() function, which determines the position of substrings inside strings
Index manipulation: Learning to use string indices as well as manipulate character position within long strings can be achieved by solving this exercise.
Strategy:
The solution to this problem involves using the Java String class method called indexOf(). The specified substrings or words appear as indexes through the use of this method when they occur for the first time. When there is no finding the word, the method returns -1.
Here’s a simple strategy to follow:
The system can accept two components: a sentence or a specific word.
Both the sentence and the word are converted into lowercase for a case-insensitive search function.
Determine the word position through the indexOf() method execution.
The program will return the index value if successful or will show that the word was absent from the sentence.
The method enables you to execute word searches while developing index-handling expertise and increasing your comprehension of string methods in Java.
Word Search
Challenge: Create an application that detects specified words inside a grid and then emphasizes their locations. This type of task belongs to classic java programming challenges that test multidimensional thinking and algorithmic precision.
Concepts Covered:
Multidimensional arrays: This lesson demonstrates how to manipulate two-dimensional arrays (grids) in Java because they form the basis for managing multiple data points distributed through rows and columns. This is a vital part of java programmer skills, particularly in structured data scenarios.
Pattern matching: Pattern matching demands the development of algorithms for grid exploration through multiple directions to find specific word patterns. These kinds of java coding challenges are excellent for refining control flow logic and problem-solving abilities.
Strategy: A nested loop system traverses the grid for horizontal, vertical, and diagonal movements as the main strategy for the solution. Each grid cell requires a full examination to determine if any word begins at that position facing any of the four directions. The examination of each possible word beginning cell should inspect all directions, including horizontal left to right sequences and vertical top to bottom paths alongside the two diagonal paths.
Here’s a step-by-step approach:
Set Up the Grid: The grid requires a two-dimensional array representation, which can be executed with Java through char[][] format.
Word Search Directions: Each grid cell needs a check on whether the word begins at this position in every direction, from top-left to bottom-right and bottom-left to top-right. You should highlight the identified word (through storage of coordinates or swapping letter characters) when it gets detected.
Nested Loops: The iteration process over all rows and columns should use nested loops. The adjacent cells in each of the four directions need to be checked starting from this position.
Efficiency: The algorithm should check words in directions based on their complete fit only (avoid downward checks if the word exceeds row length).
A combination of nested loops with effective direction-oriented searches enables you to solve grid-based pattern matching tasks. Working on this challenge helps Java programmers develop skills in word search algorithms using multidimensional arrays, which enhances their expertise with arrays and pattern-matching complexity.
Anagrams
Challenge: Create an application that detects specified words inside a grid and then emphasizes their locations. This is a classic example of java coding challenges that sharpens logical reasoning and deepens understanding of string operations and sorting logic.
Concepts Covered:
Sorting algorithms: In this assignment, you will be introduced to sorting techniques that help evaluate character sequences in two strings. Using sorting, you can confirm that two words have matching characters apart from their frequencies. This practice strengthens your java programmer skills and helps in handling java coding problems and solution with classes, especially when paired with string-based algorithms.
Array manipulation: In this task, you will also deal with arrays by converting strings to character arrays before performing sorting methods and comparison tasks.
Strategy: The resolution of this task involves transforming both input words into character arrays before executing alphabetical sorting operations. The word anagram can be confirmed if both words have matching ordered character arrays, making this a valuable java programming challenge for logic-building.
Here’s a step-by-step strategy to follow:
Convert the Strings to Character Arrays: Start by converting both input strings into character arrays. This can be done using the toCharArray() method.
Sort the Arrays: Use Java’s built-in sorting method, Arrays.sort(), to sort both arrays. Sorting will arrange the characters in alphabetical order.
Compare the Arrays: To finish, evaluate the arrays' equality by applying Arrays.equals(). The arrays match each other only when the two words share an anagram relationship.
Edge Case Handling: Check for different string lengths and non-alphabetic characters since these conditions lead to strings being unable to match as anagrams.
The approach solves anagram-checking problems in an efficient and clean manner. The combination of sorting and array manipulation provides both the solution to this problem and additional practice in Java sorting algorithms and array operations.
Pangrams
Challenge: Create a program that determines whether a provided sentence contains each letter from the alphabet in a pangram way. This forms part of java coding challenges that help solidify logic and character handling.
Concepts Covered:
Character validation: The validation challenge requires java developers to test every character in a sentence for the presence of all 26 English alphabet letters.
Data structures (Sets): You need a HashSet as data structure to track unique characters because it keeps duplicate removal easy when counting letters. Using sets proves beneficial because sets naturally take care of any repeated entries.
Strategy:
The best solution to address this problem involves employing a HashSet to monitor all letters as you read the sentence. Each character you encounter should be added to the set during processing. The set contains all 26 letters, which indicates that the sentence functions as a pangram.
Here’s a step-by-step strategy to follow:
Convert to Lowercase: The following procedure outlines how to tackle this problem: The first step consists of converting the entire sentence into lowercase format. The conversion to lowercase handles both capital and lowercase letters during checks, so "A" equals "a".
Track Letters with a HashSet: A HashSet tracks each letter. Now, examine every character in the sentence. The program adds alphanumeric characters (from 'a' to 'z') to a HashSet during every iteration. The HashSet software detects duplicated letters in the input, so it stores only distinctive letters.
Check Set Size: The HashSet should display 26 items to confirm proper processing of the sentence because there are 26 distinct letters in the English alphabet. A sentence qualifies as a pangram when all letters of the alphabet appear in its sequence.
Edge Case Handling: The program allows you to manage edge situations where sentences either have no content or have fewer than 26 words, thus failing to qualify as pangrams.
The set-up demonstrates high efficiency since O(1) operations detect letter presence, which maintains quick response for lengthy sentences. Sets improve the overall logic because they automatically handle duplicate characters. The assignment will enhance your capabilities for Java data structure application alongside character validation functionality.
Count Vowels and Consonants
Challenge: The Java application counts vowels versus consonants within a given string.
Concepts Covered:
Character classification: Your task consists of categorizing each letter within strings into either letter class or both by following the general rules of phonetics and the alphabet.
Loop handling: The exercise needs you to implement a loop for character-by-character string traversal, as this strengthens your ability to work with strings effectively.
Strategy:
Here’s a step-by-step approach to follow:
Convert to Lowercase: The first step should transform the whole string into lowercase characters because it simplifies the handling of upper and lower-case letters.
Initialize Counters: The counting process should begin with two zero-counted markers for vowels and consonants.
Loop Through the String: A loop should scan through all characters within the string. For each character:
Check if it's a letter (using Character.isLetter()).
If it's a vowel (i.e., 'a', 'e', 'i', 'o', 'u'), increment the vowel counter.
If it's a consonant (i.e., any alphabet letter that's not a vowel), increment the consonant counter.
Edge Case Handling: During the counting process, totally disregard all characters aside from normal alphabet characters, including spaces, numbers, and punctuation marks.
Display the Result: The program finishes its task by displaying the total count of vowels and consonants from the input string.
This exercise provides you a chance to practice Java string traversal and character classification while improving your knowledge about handling loops and conditions in Java. A basic template for your code can be described as follows:
public class VowelConsonantCounter { public static void main(String[] args) { String input = "Hello World!"; int vowels = 0, consonants = 0; // Convert the string to lowercase for case-insensitive comparison input = input.toLowerCase(); for (int i = 0; i < input.length(); i++) { char ch = input.charAt(i); // Check if the character is a letter if (Character.isLetter(ch)) { // Check for vowels if (ch == 'a' || ch == 'e' || ch == 'i' || ch == 'o' || ch == 'u') { vowels++; } else { consonants++; } } } // Output the result System.out.println("Vowels: " + vowels); System.out.println("Consonants: " + consonants); } }
Through this challenge, you will gain a better comprehension of Java character classification methods and loop processing techniques, which are important skills for string manipulation.
Number Reversal
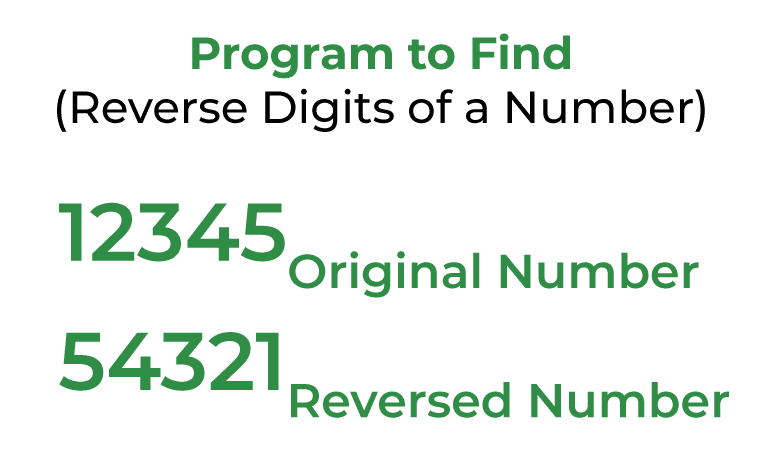
Challenge: Write a program to reverse the digits of a given number.
Concepts Covered:
Mathematical operations: This challenge will require using mathematical operations such as the modulus operator (%) and integer division (/) to extract and manipulate digits.
Looping techniques: You'll practice using loops to iteratively process the digits of the number and reconstruct it in reverse order.
Here's a basic example of how your code might look:
import java.util.Scanner;public class NumberReversal { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print("Enter a number: "); int num = scanner.nextInt(); int reversedNum = 0; // Handle negative numbers int sign = num < 0 ? -1 : 1; // Preserve the sign of the number num = Math.abs(num); // Work with absolute value for reversal // Reversing the number while (num != 0) { int digit = num % 10; // Get the last digit reversedNum = reversedNum * 10 + digit; // Append the digit num = num / 10; // Remove the last digit } // If the original number was negative, make the result negative reversedNum *= sign; System.out.println("Reversed Number: " + reversedNum); } }
Armstrong Numbers
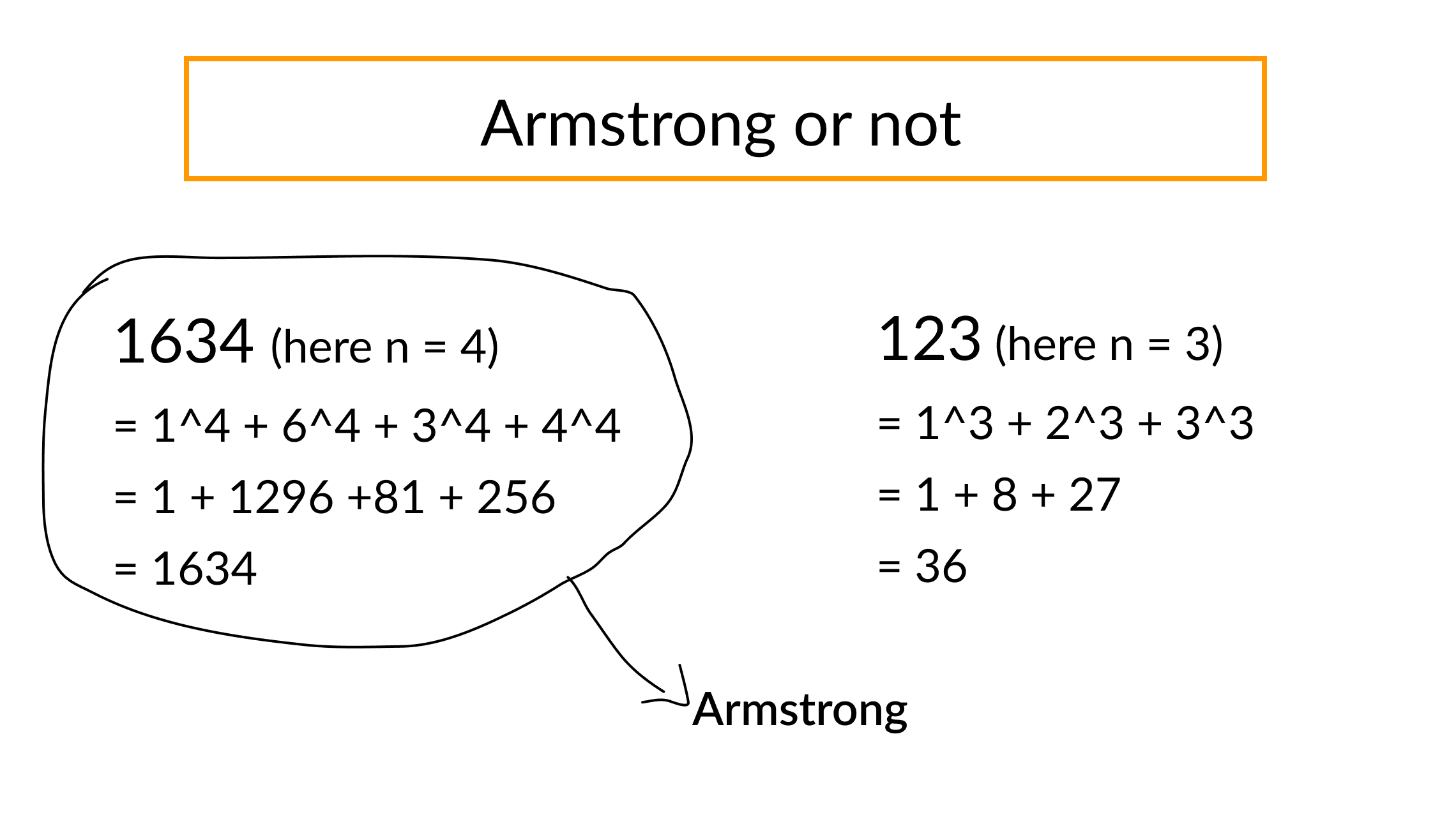
Challenge: Check if a given number is an Armstrong number (sum of cubes of its digits equals the number).
Concepts Covered:
Mathematical logic: This challenge involves understanding the concept of Armstrong numbers, which requires manipulating the digits of a number using mathematical operations like modulus and division. You'll also practice using mathematical logic to verify the Armstrong number condition.
Loop iteration: Through looping mechanisms, you will step through every digit while extracting numbers to calculate cubes for summing the results.
Here's an example of code implementation:
import java.util.Scanner;public class ArmstrongNumber { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print("Enter a number: "); int num = scanner.nextInt(); int originalNum = num; int sum = 0; // Loop through each digit and calculate the sum of cubes while (num != 0) { int digit = num % 10; // Extract the last digit sum += Math.pow(digit, 3); // Cube the digit and add to sum num /= 10; // Remove the last digit } // Check if the sum of cubes is equal to the original number if (sum == originalNum) { System.out.println(originalNum + " is an Armstrong number."); } else { System.out.println(originalNum + " is not an Armstrong number."); } } }
Product Maximiser
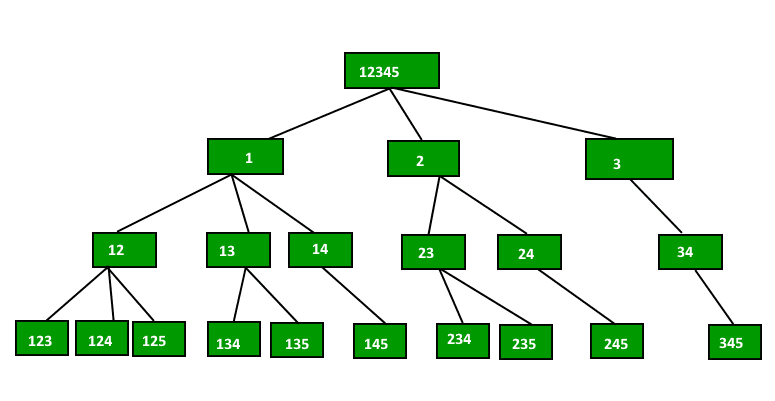
Challenge: Create a Java program to identify the greatest possible product combination between array elements. This is a key java coding challenge that reinforces array sorting and conditional logic.
Strategy:
Sort the Array: The sorting operation will produce an array structure optimized for rapid examination of maximum and minimum numeric values. The maximum product emerges either from the multiplication of the two biggest positive values or from the two most negative numbers because negative numbers multiplied together yield a positive result.
Evaluate Combinations: After sorting, the sequence becomes eligible for maximum product evaluation.
The product of the two largest positive numbers.
The product of the two smallest negative numbers could potentially lead to a larger positive value, depending on the high negativity of both numbers.
Return the Maximum Product: Determine the Bigger Result by Comparing the Two Products.
import java.util.Arrays;public class ProductMaximizer { public static void main(String[] args) { int[] nums = {1, 10, -5, 1, -100}; System.out.println("Maximum Product: " + findMaxProduct(nums)); } public static int findMaxProduct(int[] nums) { // sorted array Arrays.sort(nums); // Calculate the product of the two largest numbers (last two elements) int product1 = nums[nums.length - 1] * nums[nums.length - 2]; // Calculate the product of the two smallest numbers (first two elements) int product2 = nums[0] * nums[1]; // Return the maximum of the two products return Math.max(product1, product2); } }
Prime Number Checker
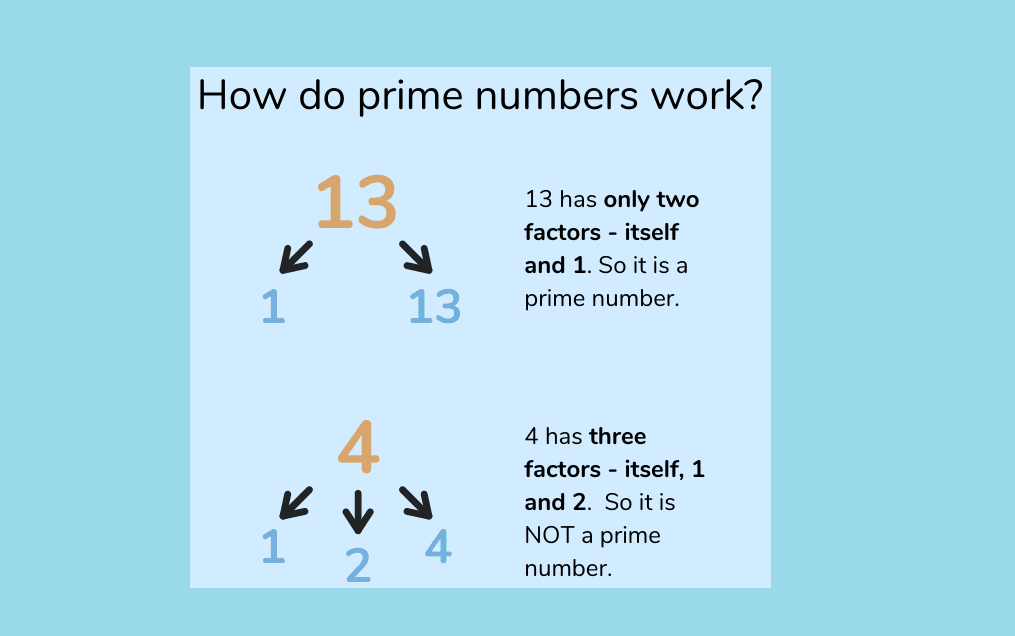
Challenge: The program must determine whether a given number is prime.
Concepts Covered:
Mathematical Optimization: Mathematical Optimization serves as the prime focus of this challenge, which demonstrates the square root method for prime number checks. The process of prime number determination requires the examination of numbers only up to a square root value rather than the original number value, which leads to radical efficiency improvements.
Conditional Loops: Loops with conditional statements will ensure you check whether numbers can divide by factors that exceed 1 and itself.
Code Implementation:
import java.util.Scanner;public class PrimeNumberChecker { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print("Enter a number: "); int num = scanner.nextInt(); if (isPrime(num)) { System.out.println(num + " is a prime number."); } else { System.out.println(num + " is not a prime number."); } } // Function to check if a number is prime public static boolean isPrime(int num) { // Edge case: numbers less than 2 are not prime if (num <= 1) { return false; } // Handle 2 as a special case if (num == 2) { return true; // 2 is the only even prime number } // If the number is even and greater than 2, it is not prime if (num % 2 == 0) { return false; } // Check divisibility from 3 to sqrt(num) int sqrt = (int) Math.sqrt(num); for (int i = 3; i <= sqrt; i += 2) { if (num % i == 0) { return false; // If divisible by i, it's not prime } } // If no divisors found, it's prime return true; } }import java.util.Scanner;public class PrimeNumberChecker { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print("Enter a number: "); int num = scanner.nextInt(); if (isPrime(num)) { System.out.println(num + " is a prime number."); } else { System.out.println(num + " is not a prime number."); } } // Function to check if a number is prime public static boolean isPrime(int num) { // Edge case: numbers less than 2 are not prime if (num <= 1) { return false; } // Handle 2 as a special case if (num == 2) { return true; // 2 is the only even prime number } // If the number is even and greater than 2, it is not prime if (num % 2 == 0) { return false; } // Check divisibility from 3 to sqrt(num) int sqrt = (int) Math.sqrt(num); for (int i = 3; i <= sqrt; i += 2) { if (num % i == 0) { return false; // If divisible by i, it's not prime } } // If no divisors found, it's prime return true; } }
Prime Factorization
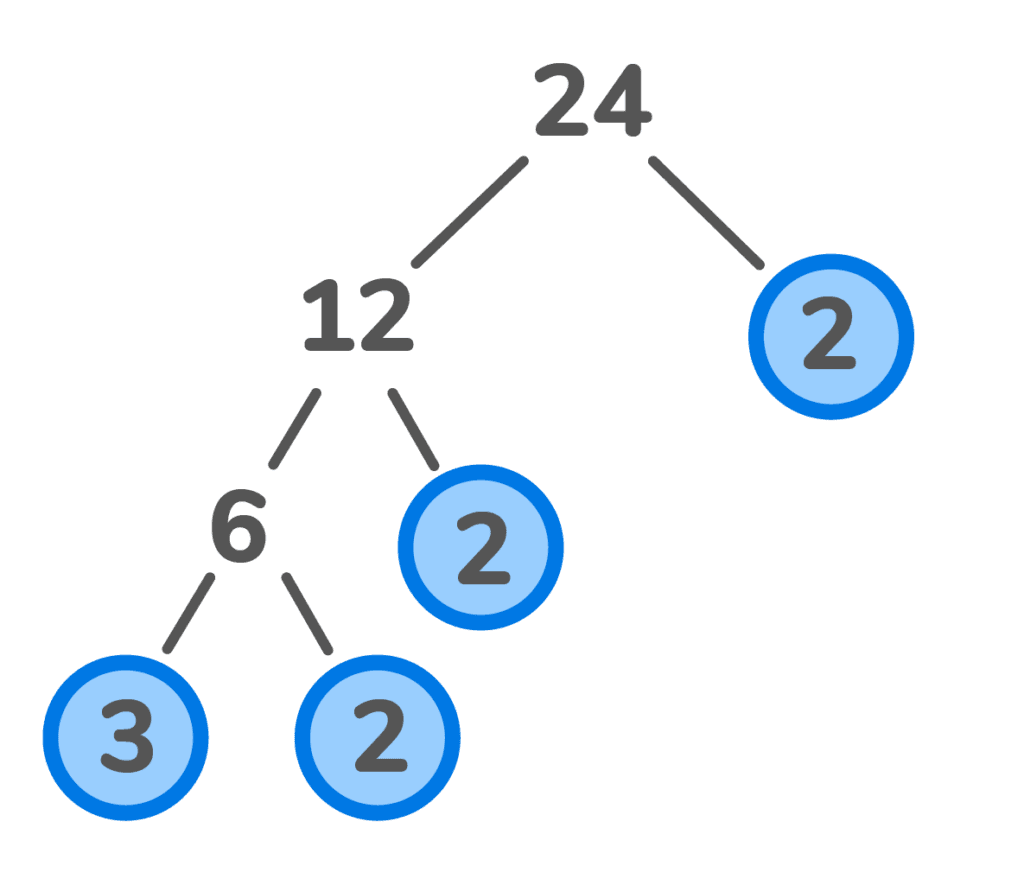
Challenge: Build a system that factors any specified number by its prime components.
Concepts Covered:
Prime Checking: Prime Checking functions as a test to identify prime numbers, allowing prime factor decomposition of entered numbers.
Iterative Decomposition: The process involves continuous division of the number with its smallest prime factors until the number reaches value 1. The iterative function will split a number into its basic prime elements.
Code Implementation:
import java.util.ArrayList;import java.util.List;import java.util.Scanner;public class PrimeFactorization { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print("Enter a number: "); int num = scanner.nextInt(); List<Integer> primeFactors = getPrimeFactors(num); System.out.print("Prime factors of " + num + ": "); for (int factor : primeFactors) { System.out.print(factor + " "); } } // Function to get prime factors of a number public static List<Integer> getPrimeFactors(int num) { List<Integer> factors = new ArrayList<>(); // Handle the factor of 2 separately to optimize for even numbers while (num % 2 == 0) { factors.add(2); num /= 2; } // Check for odd factors from 3 upwards for (int i = 3; i * i <= num; i += 2) { while (num % i == 0) { factors.add(i); num /= i; } } // If the remaining number is a prime greater than 2 if (num > 2) { factors.add(num); } return factors; } }
Summation
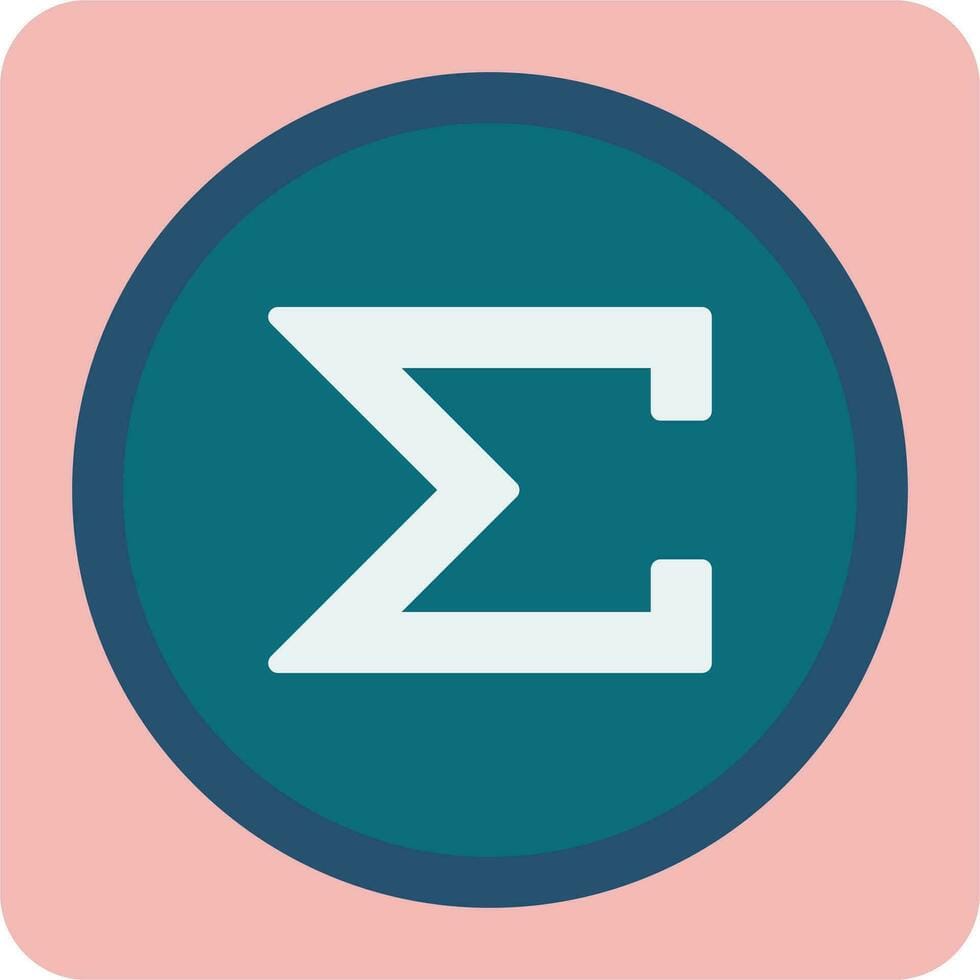
Challenge: Java development requires building software to obtain the total integer numbers in a specified range.
Concepts Covered:
Looping Structures: The problem demands a looping structure that completes range numbers and then adds them together through its loops.
Mathematical Calculations: The use of arithmetic sum formula enables mathematical optimization by removing the need for a looping structure.
Code Implementation:
import java.util.Scanner;public class Summation { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print("Enter the starting number (m): "); int m = scanner.nextInt(); System.out.print("Enter the ending number (n): "); int n = scanner.nextInt(); // Calculate the sum using a loop int sum = calculateSumWithLoop(m, n); System.out.println("The sum of integers from " + m + " to " + n + " is: " + sum); } // Function to calculate sum using a loop public static int calculateSumWithLoop(int m, int n) { int sum = 0; for (int i = m; i <= n; i++) { sum += i; } return sum; } }
Advancing Your Career with Java
Learning to solve Java programming puzzles leads to complete skill improvement, which directly opens many career possibilities. The versatility of Java as a programming language makes it an appealing choice for beginners due to its extensive use in the market, thus providing seasoned professionals opportunities to shift toward advanced development areas in their careers.
Software Development –
Java establishes fame due to its reliable performance and capacity to scale applications, therefore becoming a perfect choice for extensive system development. Java mastery allows you to enter the software development field to create software systems at various scales, from enterprise applications to cloud-based solutions.
Data Analysis –
Java’s advanced processing method enables its widespread application for scientific computing and data analysis tasks. The handle of Java datasets, combined with libraries including Apache Hadoop and Apache Spark, creates a perfect environment for big data and machine learning applications.
Mobile App Development –
The Java programming framework powers all Android application development operations. Excellence in Java programming practice allows you to embark on mobile app development as an Android app creator who reaches millions of users.
The online Java programming platform Codeyoung delivers organized courses that implement custom learning approaches that help students understand fundamental principles completely. Java programming courses enable students to progress from beginner to advanced levels through practical learning sessions guided by experts who establish their knowledge base while building skills to address complex code problems.
The online platform Codeyoung offers a guided path for students of all levels. Learners build competence through structured modules that center around Java coding challenges, practical projects, and real-time feedback. With each lesson, students advance their skills in a logical progression—from foundational concepts to advanced application—supported by mentors who focus on real-world outcomes.
Java Programming Challenges – FAQs
How can learning basic Java programming codes help beginners tackle Java programming challenges effectively?
Beginners who master simple Java programming codes acquire essential understanding about loops alongside arrays together with conditionals and methods. The basic tools that build foundations for solving advanced problems constitute essential building blocks. The successful solution of complicated Java programming challenges requires beginner programmers to master these fundamental coding elements.
What are some practical Java coding problems and solutions with classes for beginners to practice?
Examples include:
Prime Number Checker: The code provides a basic functionality to determine whether a number is prime.
Word Search: The program conducts letter word lookups in rectangular layout patterns.
Anagram Checker: A code implementation that performs anagram checking by verifying if two words hold the same character elements independent of arrangement order.
This sort of practice enables novices to discover methods for structuring programs with classes while teaching them to employ object-oriented design principles and work on Java coding problems and solutions.
Why is it important for beginners to practice coding skills in Java exercises to improve their programming skills?
Coding challenge practice during normal sessions enables novice programmers to enhance their ability to solve problems and develop their logical thinking capabilities. Becoming familiar with fundamental Java principles happens through this practice, including syntax knowledge and data structures together with algorithms. The solution of various problems provides learners the opportunity to divide big projects into logical segments and develop their ability to produce code that is both efficient and easy to understand through regular Java exercises.
How can Java programming practice exercises help students build essential Java programming skills?
Students who engage in regular Java programming practice exercises strengthen their comprehension of critical programming principles, including loops, conditionals, and object-oriented design and algorithms. Students learn to debug code while they develop optimization skills in coding, which prepares them for actual software development practice and enhances their Java programming skills.
How can understanding Java programming uses motivate beginners to tackle coding challenges with confidence?
The wider knowledge of Java applications from web development to mobile app development and scientific computing allows beginners to recognize how mastering Java brings them closer to actual project work. The students gain an intense drive that pushes them to face additional coding challenges because of their developing passion for learning.